Hello Friends I am back again with a amazing tutorial to create a calculator in c++ language.We'll make a simple calculator that will do the below given things in order:
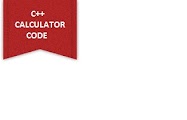
We’re going to get a number from the user and return it back to the caller. Thus, the function prototype would look like this:
[code]
int getUserInput();
[/code]
In our calculator , the calculateResult() function will need to take 3 pieces of input: Two numbers and a mathematical operator. We should already have all three of these by the time we get to the point where this function is called, so these three pieces of data will be function parameters. The calculateResult() function will calculate the result value, but it does not display the result itself. Consequently, we need to return that result as a return value so that other functions can use it.
Given that, we could write the function prototype like this:
[code]
int calculateResult(int input1, int op, int input2);
[/code]
Now we want mathematical operation from user, we can do that as follows:
[code]
int getMathematicalOperation()
{
std::cout << "Please enter which operator you want (1 = +, 2 = -, 3 = *, 4 = /): "; int op; std::cin >> op;
/*Note that we are ignoring the possibility of user entering an invalid character, this will be covered in some other tutorial*/
return op;
}
[/code]
Finally, the last step is to connect up the inputs and outputs of each task in whatever way is appropriate. For example, you might send the output of calculateResult() into an input of printResult(), so it can print the calculated answer. This will often involve the use of intermediary variables to temporarily store the result so it can be passed between functions. For example:
[code]
// result is a temporary value used to transfer the output of calculateResult()
// into an input of printResult()
int result = calculateResult(input1, op, input2); // temporarily store the calculated result in result
printResult(result);
[/code]
This tends to be much more readable than the alternative condensed version that doesn’t use a temporary variable:
[code]
printResult( calculateResult(input1, op, input2) );
[/code]
At this point with the help of above info you should be able to create the calculator yourself.But don't worry if you are a beginner in c++ i am here to make your life easier.A fully completed code of calculator is given below:
[code]
// #include "stdafx.h" // uncomment if using visual studio
#include
int getUserInput()
{
std::cout << "Please enter an integer: "; int value; std::cin >> value;
return value;
}
int getMathematicalOperation()
{
std::cout << "Please enter which operator you want (1 = +, 2 = -, 3 = *, 4 = /): "; int op; std::cin >> op;
// What if the user enters an invalid character?
// We'll ignore this possibility for now
return op;
}
int calculateResult(int x, int op, int y)
{
// note: we use the == operator to compare two values to see if they are equal
// we need to use if statements here because there's no direct way to convert op into the appropriate operator
if (op == 1) // if user chose addition (#1)
return x + y; // execute this line
if (op == 2) // if user chose subtraction (#2)
return x - y; // execute this line
if (op == 3) // if user chose multiplication (#3)
return x * y; // execute this line
if (op == 4) // if user chose division (#4)
return x / y; // execute this line
return -1; // default "error" value in case user passed in an invalid op
// note: This isn't a good way to handle errors, since -1 could be returned as a legitimate value
}
void printResult(int result)
{
std::cout << "Your result is: " << result << std::endl; } int main() { // Get first number from user int input1 = getUserInput(); // Get mathematical operation from user int op = getMathematicalOperation(); // Get second number from user int input2 = getUserInput(); // Calculate result and store in temporary variable (for readability/debug-ability) int result = calculateResult(input1, op, input2 ); // Print result printResult(result); } [/code]
SUBSCRIBE US AND LIKE OUR FB PAGE TO GET LATEST UPDATES.
- Gets a number from the user.
- Gets a mathematical operation from the user (+,-,*,/).
- Gets another number from the user.
- Calculates the result and store it in a variable.
- Print the result on the screen.
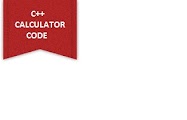
So Let's Get Into The Tutorial
We’re going to get a number from the user and return it back to the caller. Thus, the function prototype would look like this:
[code]
int getUserInput();
[/code]
In our calculator , the calculateResult() function will need to take 3 pieces of input: Two numbers and a mathematical operator. We should already have all three of these by the time we get to the point where this function is called, so these three pieces of data will be function parameters. The calculateResult() function will calculate the result value, but it does not display the result itself. Consequently, we need to return that result as a return value so that other functions can use it.
Given that, we could write the function prototype like this:
[code]
int calculateResult(int input1, int op, int input2);
[/code]
Now we want mathematical operation from user, we can do that as follows:
[code]
int getMathematicalOperation()
{
std::cout << "Please enter which operator you want (1 = +, 2 = -, 3 = *, 4 = /): "; int op; std::cin >> op;
/*Note that we are ignoring the possibility of user entering an invalid character, this will be covered in some other tutorial*/
return op;
}
[/code]
Finally, the last step is to connect up the inputs and outputs of each task in whatever way is appropriate. For example, you might send the output of calculateResult() into an input of printResult(), so it can print the calculated answer. This will often involve the use of intermediary variables to temporarily store the result so it can be passed between functions. For example:
[code]
// result is a temporary value used to transfer the output of calculateResult()
// into an input of printResult()
int result = calculateResult(input1, op, input2); // temporarily store the calculated result in result
printResult(result);
[/code]
This tends to be much more readable than the alternative condensed version that doesn’t use a temporary variable:
[code]
printResult( calculateResult(input1, op, input2) );
[/code]
At this point with the help of above info you should be able to create the calculator yourself.But don't worry if you are a beginner in c++ i am here to make your life easier.A fully completed code of calculator is given below:
[code]
// #include "stdafx.h" // uncomment if using visual studio
#include
int getUserInput()
{
std::cout << "Please enter an integer: "; int value; std::cin >> value;
return value;
}
int getMathematicalOperation()
{
std::cout << "Please enter which operator you want (1 = +, 2 = -, 3 = *, 4 = /): "; int op; std::cin >> op;
// What if the user enters an invalid character?
// We'll ignore this possibility for now
return op;
}
int calculateResult(int x, int op, int y)
{
// note: we use the == operator to compare two values to see if they are equal
// we need to use if statements here because there's no direct way to convert op into the appropriate operator
if (op == 1) // if user chose addition (#1)
return x + y; // execute this line
if (op == 2) // if user chose subtraction (#2)
return x - y; // execute this line
if (op == 3) // if user chose multiplication (#3)
return x * y; // execute this line
if (op == 4) // if user chose division (#4)
return x / y; // execute this line
return -1; // default "error" value in case user passed in an invalid op
// note: This isn't a good way to handle errors, since -1 could be returned as a legitimate value
}
void printResult(int result)
{
std::cout << "Your result is: " << result << std::endl; } int main() { // Get first number from user int input1 = getUserInput(); // Get mathematical operation from user int op = getMathematicalOperation(); // Get second number from user int input2 = getUserInput(); // Calculate result and store in temporary variable (for readability/debug-ability) int result = calculateResult(input1, op, input2 ); // Print result printResult(result); } [/code]
Uncomment the first line of the code if you are using visual studio to compile your code.
That's all friends hope you enjoyed reading the tutorial.Share this article as more as you can so that other can also read this.And don't forget to express your views via comment.
SUBSCRIBE US AND LIKE OUR FB PAGE TO GET LATEST UPDATES.
0 comments:
Post a Comment